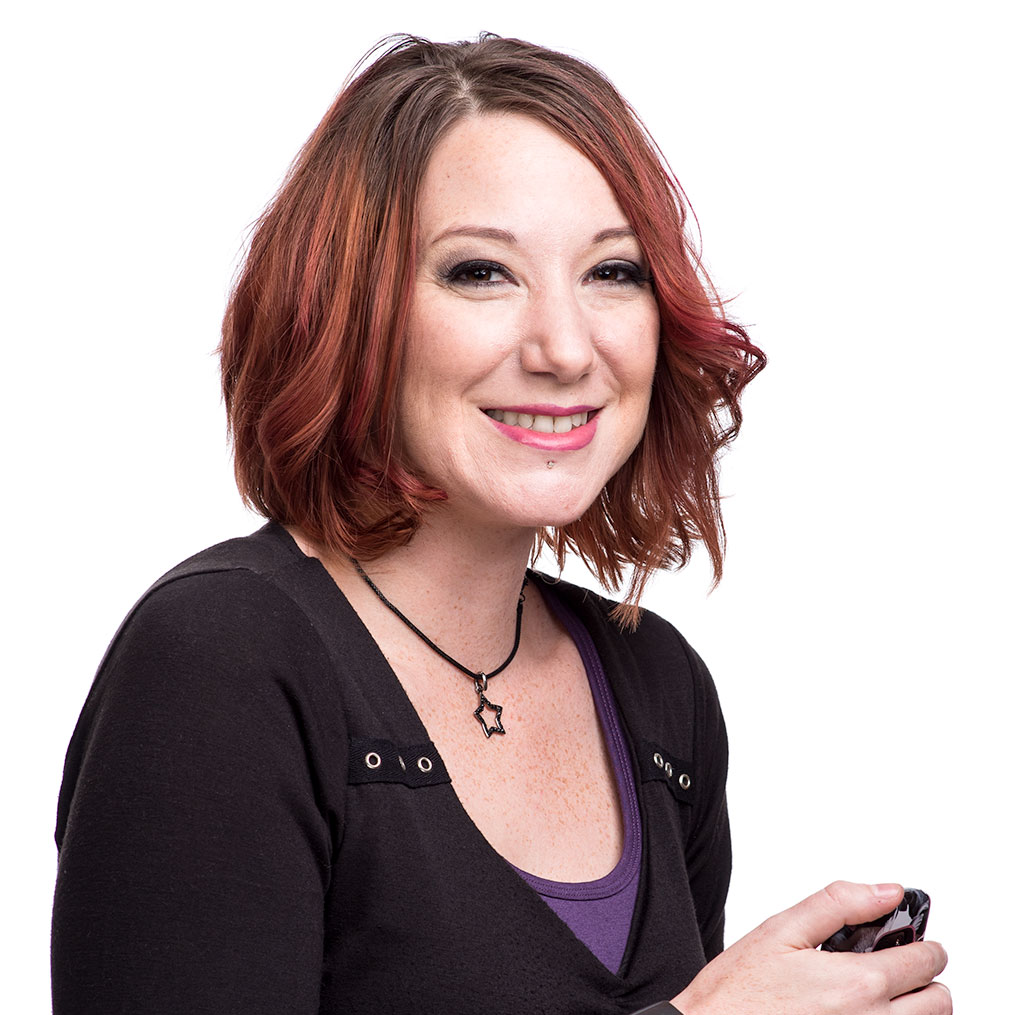
Custom Web Development |
Integrated Dashboards
Written By Tabytha Rourke
Lead Web Developer at Lee Media Group
Thank you for attending my Wordcamp KC 2018 presentation!
Learn how to create custom integrated dashboards for your WordPress sites that provide real-time analytics and other data to clients directly from their admin dashboard! You can view the presentation slides on Google Drive.
Do you need help customizing your WordPress dashboard? Contact us today!
Custom Dashboard Examples
Customize the Admin Menu
Clean up unnecessary options with remove_menu_page
add_action( 'admin_menu', 'my_remove_menu_pages', 99); function my_remove_menu_pages() { global $current_user; if( in_array('client', $current_user->roles) && !in_array('administrator', $current_user->roles) && !in_array('editor', $current_user->roles) ) { remove_menu_page( 'edit-comments.php' ); // hide comments remove_menu_page( 'tools.php' ); // hide tools remove_menu_page( 'upload.php' ); // hide media library remove_menu_page( 'profile.php' ); // hide profile link remove_menu_page( 'edit.php?post_type=shop_order' ); // hide woocommerce orders } }
Add custom options with add_menu_page
add_action( 'admin_menu', 'my_add_menu_pages', 99); function my_add_menu_pages() { global $current_user; if( in_array('client', $current_user->roles) || in_array('editor', $current_user->roles) ) { // add new custom page add_menu_page( 'My FAQs', 'My FAQs', 'clients', 'my-faqs', 'my_faqs', 'dashicons-editor-help', 50); // move Add Post to main menu add_menu_page( 'Add Post', 'Add Post', 'clients', 'post-new.php', '', 'dashicons-admin-post', 51); } }
Customize the Admin Bar
Customize the admin bar with admin_bar_menu
add_action( 'admin_bar_menu', 'custom_wp_admin_bar', 99); function custom_wp_admin_bar( $wp_admin_bar ) { $wp_admin_bar->remove_node('wp-logo'); $wp_admin_bar->remove_node('new-faqs'); $args = array( 'id' => 'lmg', // Set the ID of your custom link 'title' => 'LMG', // Set the title of your link 'href' => 'https://leemediagroup.com/lmg-cms/', // Define the destination of your link 'meta' => array( 'target' => '_blank', // Change to _blank for launching in a new window or tab 'class' => 'lmg', // Add a class to your link 'title' => 'LMG' // Add a title to your link ) ); $wp_admin_bar->add_node($args); }
Control admin bar visibility with show_admin_bar
add_action('init', 'configure_admin_bar'); function configure_admin_bar() { if ( !current_user_can('manage_options') ) { show_admin_bar('false'); } }
Control dashboard access with custom redirects
add_action('admin_init', 'hide_the_dashboard'); function hide_the_dashboard() { global $current_user; $has_access = false; if ( current_user_can('manage_options') ) $has_access = true; if ( !is_admin() || ( defined('DOING_AJAX') && DOING_AJAX ) ) $has_acces = true; if ( !$has_access ) { wp_redirect('/member-dashboard/'); exit; } }
Custom Welcome Pages
Use add_menu_page to create a new screen
add_action('admin_menu', 'welcome_screen_pages'); function welcome_screen_pages() { add_menu_page( 'Welcome', // the page title 'Welcome', // the label for the menu 'clients', // capability required to see this content 'welcome', // the page slug (admin.php?page=welcome) 'welcome_screen_content', // the function that creates the content 'dashicons-admin-home', // icon to display in the menu 1 // menu position (we want this to be at the top) ); }
Populate your new page with content
function welcome_screen_content() { global $current_user; ?>
Welcome Back,
This is where you will manage your new site.
Site Traffic:
Display Top Pages
$results = $analytics->data_ga->get( 'ga:' . $google_profile_id, '30daysAgo', 'yesterday', 'ga:pageviews', array( 'max-results' => 10, 'sort' => '-ga:pageviews', 'dimensions' => 'ga:pageTitle, ga:pagePath', 'filters' => 'ga:pagePathLevel1!=/' )); $pageviews = $results->getRows(); ?>
Top Pages
# | Page | Pageviews |
---|---|---|
Display Views by City
By City
Display Views by Device
By Device
Facebook Insights Integration
- Learn more about the Facebook Graph API
- Register and Configure an App
- Facebook PHP SDK
- Extended Access Page Tokens
- Graph API Explorer
Setup The API
(The quickstart guide will help you get to this point.)
require_once get_stylesheet_directory().'/Facebook/autoload.php'; // change path as needed $fb = new \Facebook\Facebook([ 'app_id' => 'your app id', 'app_secret' => 'your app secret', 'default_graph_version' => 'v2.10' ]); $account_page_id = 'your page id'; $account_token = 'your account token';
Get Page View Graph data
$start = date('F j', strtotime('-7 days')); $end = date('F j, Y'); $current_page_views = $fb->get('/'.$account->page_id.'/insights/page_views_total?date_preset=last_7d', $account->token ); $current_views=$current_page_views->getGraphEdge()->asArray(); $week_total = 0; $labels = array("'".$current_views[0]['values'][0]['end_time']->format('F j, Y')."'"); $values = array($current_views[0]['values'][0]['value']); foreach ( $current_views[0]['values'] as $days ) { $labels[] = "'".$days['end_time']->format('F j, Y')."'"; $values[] = $days['value']; $week_total+= $days['value']; $track = 0; } $prev_start = strtotime('-14 days'); $prev_end = strtotime('-8 days'); $prev_week_views = $fb->get('/'.$account->page_id.'/insights/page_views_total?until='.$prev_end.'&since='.$prev_start, $account->token ); $prev_views=$prev_week_views->getGraphEdge()->asArray(); $prev_total = 0; foreach ( $prev_views[0]['values'] as $days ) { $prev_total+= $days['value']; $track = 0; } $views_change = ( ( $week_total - $prev_total ) / $prev_total ) * 100; if ( $views_change > 0 ) $change_format = ' '.ceil($views_change).'%'; else $change_format = ' '.ceil($views_change).'%';
Display the Page View graph
Page Views to
Total Page Views
Display Page Likes graph
$current_page_likes = $fb->get('/'.$account->page_id.'/insights/page_fan_adds?date_preset=last_7d', $account->token ); $current_likes=$current_page_likes->getGraphEdge()->asArray(); $week_total = 0; $labels = array("'".$current_likes[0]['values'][0]['end_time']->format('F j, Y')."'"); $values = array($current_likes[0]['values'][0]['value']); foreach ( $current_likes[0]['values'] as $days ) { $labels[] = "'".$days['end_time']->format('F j, Y')."'"; $values[] = $days['value']; $week_total+= $days['value']; $track = 0; } $prev_start = strtotime('-14 days'); $prev_end = strtotime('-8 days'); $prev_page_likes = $fb->get('/'.$account->page_id.'/insights/page_fan_adds?until='.$prev_end.'&since='.$prev_start, $account->token ); $prev_likes=$prev_page_likes->getGraphEdge()->asArray(); $prev_total = 0; foreach ( $prev_likes[0]['values'] as $days ) { $prev_total+= $days['value']; $track = 0; } $views_change = ( ( $week_total - $prev_total ) / $prev_total ) * 100; if ( $views_change >= 0 ) $change_format = ' '.ceil($views_change).'%'; else $change_format = ' '.ceil($views_change).'%'; ?>
Page Likes to
New Page Likes
Audience by Gender
$age_demographics = $fb->get('/'.$account->page_id.'/insights/page_fans_gender_age', $account->token ); $age_gender=$age_demographics->getGraphEdge()->asArray(); $male = 0; $female = 0; $unspecified = 0; foreach ( $age_gender[0]['values'][1]['value'] as $label => $value ) { $label_pieces = explode('.', $label); if ( $label_pieces[0] == 'F' ) $female += $value; else if ( $label_pieces[0] == 'M' ) $male += $value; else $other += $value; } ?>
By Gender
Audience by Age
$age_demographics = $fb->get('/'.$account->page_id.'/insights/page_fans_gender_age', $account->token ); $age_gender=$age_demographics->getGraphEdge()->asArray(); $age_groups = array(); $age_labels = array(); foreach ( $age_gender[0]['values'][1]['value'] as $label => $value ) { $label_pieces = explode('.', $label); $age_groups[$label_pieces[1]] += $value; if ( !in_array($label_pieces[1], $age_labels) ) $age_labels[] = "'".$label_pieces[1]."'"; } ?>
By Age
Helpful Plugins
- Members by Justin Tadlock
- Client Dash
- Admin Bar & Dashboard Access Control
- Absolutely Glamorous Custom Admin
- Google Analytics for WordPress by Monster Insights
Need help setting up a custom WordPress website? Contact us today!