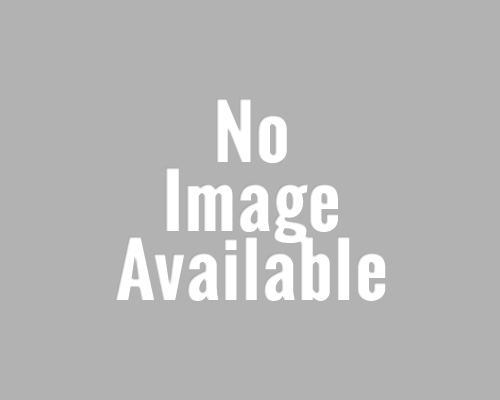
WordPress |
Organizing Your Site With Custom Post Types
Written By Tabytha Rourke
Lead Web Developer at Lee Media Group
Slideshow
Registering your new Custom Post Type
<?php function my_custom_post_types() { $args = array( 'label' => 'Movies', 'taxonomies' => array('category'), 'public' => true, 'exclude_from_search' => true, 'show_ui' => true, 'show_in_menu' => true, 'rewrite' => array('slug'=>'movies'), 'capability_type' => 'post', 'has_archive' => true, 'hierarchical' => true, 'has_archive' => true, 'menu_position' => null, 'supports' => array('title','editor','author','thumbnail') ); register_post_type( 'movies', $args ); } add_action( 'init', 'my_custom_post_types' ); ?>
Adding Custom Taxonomy to Custom Post Type
This code needs to be added right under register_post_type.
$labels = array{ 'name' => _x( 'Genre', 'taxonomy general name'), 'singular_name' => _x( 'Genre', 'taxonomy singular name'), 'search_items' => _x( 'Search Genres'), 'popular_items' => _x( 'Popular Genres'), 'all_items' => _x( 'All Genres'), 'parent_item' => null, 'parent_item_colon' => null, 'edit_item' => _x( 'Edit Genre'), 'update_item' => _x( 'Update Genre'), 'add_new_item' => _x( 'Add Genre'), 'new_item_name' => _x( 'New Genre Name'), 'seperate_items_with_commas' => _x( 'Seperate genres with commas'), 'add_or_remove_items' => _x( 'Add or remove genres'), 'choose_from_most_used' => _x( 'Choose from the most used genres'), 'not_found' => _x( 'No genres found.'), 'menu_name' => _x( 'Genres'), ); $args = array( 'hierarchical' => false, 'labels' => $labels, 'show_ui' => true, 'show_admin_column' => true, 'query_var' => true, 'rewrite' => array( 'slug', 'genre' ), ); register_taxonomy( 'genre', 'movies', $args ); }
Single Post Template
<?php /** * The template for displaying all single posts and attachments */ get_header(); ?> <div id="page" role="main"> <?php while ( have_posts() ) : the_post(); ?> <header> <h2 class="entry-title"><?php the_title(); ?></h2> </header> <?php do_action( 'foundationpress_post_before_entry_content' ); ?> <div class="entry-content"> <?php if ( has_post_thumbnail() ) : the_post_thumbnail(); endif; ?> <?php the_content(); ?> </div> <footer> <?php wp_link_pages( array('before' => '<nav id="page-nav"><p>' . __( 'Pages:', 'foundationpress' ), 'after' => '</p></nav>' ) ); ?> <p><?php the_tags(); ?></p> </footer> <?php endwhile;?> </div> <?php get_footer(); ?>
Archive Template
<?php /** * The template for displaying archive pages * * Used to display archive-type pages if nothing more specific matches a query. * For example, puts together date-based pages if no date.php file exists. * * If you'd like to further customize these archive views, you may create a * new template file for each one. For example, tag.php (Tag archives), * category.php (Category archives), author.php (Author archives), etc. * * @link https://codex.wordpress.org/Template_Hierarchy */ get_header(); ?> <div id="page" role="main"> <?php if ( have_posts() ) : ?> <?php /* Start the Loop */ ?> <?php while ( have_posts() ) : the_post(); ?> <?php get_template_part( 'template-parts/content', get_post_format() ); ?> <?php endwhile; ?> <?php else : ?> <?php get_template_part( 'template-parts/content', 'none' ); ?> <?php endif; // End have_posts() check. ?>= </div> <?php get_footer(); ?>
Content Template
<?php /** * The default template for displaying content * * Used for both single and index/archive/search. */ ?> <div id="post-<?php the_ID(); ?>" <?php post_class('blogpost-entry'); ?>> <header> <h2><a href="<?php the_permalink(); ?>"><?php the_title(); ?></a></h2> </header> <div class="entry-content"> <?php the_excerpt( __( 'Continue reading...', 'foundationpress' ) ); ?> <a href="<?php the_permalink(); ?>" class="button" style="float: right; padding: 10px; font-size: 20px!important;">Read More</a> </div> <footer> <?php $tag = get_the_tags(); if ( $tag ) { ?><p><?php the_tags(); ?></p><?php } ?> </footer> <hr /> </div>